JS Library
Login with Zip
Give your shoppers the option to Login to your website using Zip and pay seamlessly.
For all 'Login with Zip' flows, the customer is redirected to the Zip sign in to approve recurring payments. This is handled in part by the Zip JS library below.
The script can be added anywhere in the body or head and can be loaded asynchronously.
Sandbox:
<script src="https://zip.co/static/lib/js/checkouts-zip-sdk-checkout-js-sand/zip.checkout.sdk.min.js"></script>
Production:
<script src="https://zip.co/static/lib/js/checkouts-zip-sdk-checkout-js-prod/zip.checkout.sdk.min.js"></script>
Loading Zip buttons and Widgets
At various stages in the customer journey you will need to display the Zip call to action buttons or widgets.
Configuration
Below is an example of how to configure your Zip login or sign up buttons.
ClientId
Zip will provide this.
redirectUri
This should be a merchant server side endpoint. This is where the customer is redirected when they click the login / sign-up button. From there you will make a server to server /authorize API request to Zip and we will return the Zip login form.
returnUri
This is the address the customer will be returned after login. If omitted the customer will instead be sent back to your redirectUri.
You must declare your redirectUri
Your redirectUri must be provided to Zip for each environment to be added to our allowlist.
access_token
After the Zip customer logs in, you will create a token server side. If you then pass the access_token back to the font end JS in one of the 2 ways detailed below, user data can be captured (customer name, mobile, email), user accountID for charging and the Zip payment widgets will automatically load the customer account type and balance.
This is not required for the Login or signup buttons, but is needed to show the customer account type and balance on the payment option / account widgets or to pull customer information for account creation.
Pass the access_token in script
zip.auth.init({
accessToken: 'accessToken',
})
Put the access_token in a cookie
export const ZIP_AUTH_ACCESS_TOKEN_COOKIE_KEY = 'zip-auth-access-token';
export const ZIP_AUTH_STATE_COOKIE_KEY = 'zip-auth-state';
export const ZIP_AUTH_CODE_VERIFIER_KEY = 'zip-auth-code-verifier';
export const ZIP_AUTH_RETURN_URI_COOKIE_KEY = 'zip-auth-return-uri';
Example
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', (event) => {
zip.auth.init({
clientId: '<client_id>',
redirectUri: '<redirect_uri>',
// The return URL is the url the page need to redirect to after authneitcation.
returnUri: '<return_uri>',
accessToken: '<access_token>',
onSuccess: (user) => {
// when authentication is successful
console.log(user);
// call api to save token including refresh token and token id
},
onError: (error) => {
// when an error occurs
console.log(error);
},
});
});
</script>
Before customer login
Initially you will load either the login or sign-up button, which can be loaded by simply including the below div tags.
Login button
<div id="zip-login"></div>

Sign up button
<div id="zip-signup"></div>

After customer login
After a customer has successfully logged in to Zip and you have generated your customer account and token, you can then use your customer access token to load the account or payment options widgets instead. These will pull customer account information used for charging (accountId) and contain a call to action allowing the customer to 'change' their selected account.
Configuration additions
- Add access token
- Map user data in onSuccess function
- Add onAccountSelected handler function to capture the change when a customer uses the 'change' call to action on the payment or account widget.
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', (event) => {
zip.auth.init({
clientId:'<client_id>',
redirectUri: '<redirect_uri>',
// The return URL is the url the page need to redirect to after authneitcation.
returnUri: '<return_uri>',
accessToken: '<access_token>',
onSuccess: (user) => {
// when authentication is successful
console.log(user);
document
.getElementsByName('firstname')[0]
.setAttribute('value', user.given_name);
document
.getElementsByName('lastname')[0]
.setAttribute('value', user.family_name);
document.getElementsByName('email')[0].setAttribute('value', user.email);
},
onError: (error) => {
// when an error occurs
console.log(error);
},
onAccountSelected: (account) => {
// when account been selected / changed
console.log(account);
},
});
});
</script>
Account widget
<div id="zip-account"></div>
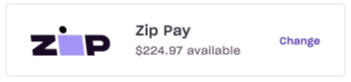
Payment option
The Zip payment option can be loaded prior to customer login too, but will not show the account balance or 'change' link. You will want to load in this way at checkout for customers who are already members on your site, but have not yet paid or logged in via Zip.
<div id="zip-payment-option"></div>
Before linking
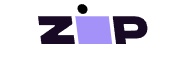
After linking
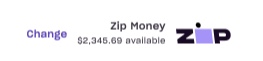
Accessing customer data
As long as your access_token was passed in the Zip init function, you will be able to access user, account and token info as below:
Get access token from front-end
const accessToken = zip.auth.accesss_token;
Get account id from front-end
const accountId = zip.auth.accountId;
Customer data
const firstName = user.given_name;
const lastName = user.family_name
const phone = user.phone_number;
const email = user.email;
Redirect to Zip
Now that the customer can be to redirected onClick to your 'redirectUri', you will need to make some server side API calls to have the user session redirect to Zip
Updated 10 months ago